/************************
** common.js [Write file text]
************************/
$(function () {
// lock control
var lock_html = '';
lock_html += '
\n';
lock_html += '
\n';
lock_html += '
\n';
lock_html += '
\n';
lock_html += '
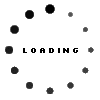
\n';
lock_html += '
\n';
lock_html += '
\n';
lock_html += '
\n';
$("body").append(lock_html);
// message control
var msg_html = '';
msg_html += '\n';
msg_html += '
\n';
$("body").append(msg_html);
$("#proccess_msg").css({ position: "fixed", bottom: "10px", right:"10px" });
// Enterで更新禁止
$("input").keypress(function (ev) {
if ((ev.which && ev.which === 13) || (ev.keyCode && ev.keyCode === 13)) {
return false;
} else {
return true;
}
});
// スクロールのオフセット値
var offsetY = -10;
// スクロールにかかる時間
var time = 500;
// ページ内リンクのみを取得
try {
$('a[href^=#]').click(function () {
// 移動先となる要素を取得
var target = $(this.hash);
if (!target.length) return;
// 移動先となる値
var targetY = target.offset().top + offsetY;
// スクロールアニメーション
$('html,body').animate({ scrollTop: targetY }, time, 'swing');
// ハッシュ書き換えとく
window.history.pushState(null, null, this.hash);
// デフォルトの処理はキャンセル
return false;
});
} catch (e) { console.log(e.message);}
// FullScreen
$(".FullScreen-ON").show();
$(".FullScreen-OFF").hide();
});
function alert_delay(msg, delay) {
if (!delay) delay = 100;
setTimeout(function () { alert(msg); }, delay);
}
/************
Open and Close
************/
// WOpen
function WOpen(url, name, w, h) {
if (!(w) && !(h)) {
w = Math.round(screen.width * 0.99);
h = Math.round(screen.height * 0.95);
window.open(url, name, 'width=' + String(w) + ', height=' + String(h) + ', left=0, top=0, menubar=no, toolbar=no, scrollbars=yes, location=no, status=no, resizable=yes');
} else if (!(w)) {
w = Math.round(screen.width * 0.99);
window.open(url, name, 'width=' + String(w) + ', height=500, left=0, top=0, menubar=no, toolbar=no, scrollbars=yes, location=no, status=no, resizable=yes');
} else {
window.open(url, name, 'width=' + String(w) + ', height=' + String(h) + ', menubar=no, toolbar=no, scrollbars=yes, location=no, status=no, resizable=yes');
}
}
// WClose
function WClose() {
var nvua = navigator.userAgent;
if (nvua.indexOf('MSIE') >= 0) {
if (nvua.indexOf('MSIE 5.0') == -1) {
top.opener = '';
}
}
else if (nvua.indexOf('Gecko') >= 0) {
top.name = 'CLOSE_WINDOW';
wid = window.open('', 'CLOSE_WINDOW');
}
top.close();
//(window.open('', '_top').opener = top).close();
return false;
}
// TimerLink
function TimerLink(ms, lnk) {
setTimeout(function () {
window.top.location.href(lnk);
}, ms);
}
// FullScreen
function FullScreen(e) {
if (!document.fullscreenElement && !document.mozFullScreenElement && !document.webkitFullscreenElement && !document.msFullscreenElement ) { // current working methods
if (document.documentElement.requestFullscreen) {
document.documentElement.requestFullscreen();
} else if (document.documentElement.msRequestFullscreen) {
document.documentElement.msRequestFullscreen();
} else if (document.documentElement.mozRequestFullScreen) {
document.documentElement.mozRequestFullScreen();
} else if (document.documentElement.webkitRequestFullscreen) {
document.documentElement.webkitRequestFullscreen(Element.ALLOW_KEYBOARD_INPUT);
}
$(".FullScreen-ON").hide();
$(".FullScreen-OFF").show();
} else {
if (document.exitFullscreen) {
document.exitFullscreen();
} else if (document.msExitFullscreen) {
document.msExitFullscreen();
} else if (document.mozCancelFullScreen) {
document.mozCancelFullScreen();
} else if (document.webkitExitFullscreen) {
document.webkitExitFullscreen();
}
$(".FullScreen-ON").show();
$(".FullScreen-OFF").hide();
}
}
/************
Auto Setting
************/
// Auto Watch
// $(".watch")
$(function () {
// watch function
var wf = (function (obj) {
if (obj) {
// make time string
now = new Date();
year = now.getYear();
month = now.getMonth() + 1;
day = now.getDate();
hour = now.getHours();
minute = now.getMinutes();
second = now.getSeconds();
if (year < 1000) { year += 1900 }
if (hour < 10) { hour = '0' + hour }
if (minute < 10) { minute = '0' + minute }
if (second < 10) { second = '0' + second }
// disp
switch ($(obj).prop("tagName").toUpperCase()) {
case "INPUT":
case "TEXTAREA":
$(obj).val(year + '/' + month + '/' + day + ' ' + hour + ':' + minute + ':' + second);
break;
default:
$(obj).html(year + '/' + month + '/' + day + ' ' + hour + ':' + minute + ':' + second);
break;
}
}
});
// watch class
$(".watch").each(function () {
var target = this;
setInterval(function () { wf(target); }, 1000);
});
});
// Auto Image Opacity
// $("a img, input[type=image]")
$(function () {
$("a img, input[type=image]").each(function () {
if ($(this).hasClass("noalpha")) {
//NA
} else {
// focus and hover
$(this).focus(function () { $(this).blur(); }).hover(
function () {
// mouseover
$(this).css({ "opacity": "0.7" });
}
, function () {
// mouseout
$(this).css({ "opacity": "1" });
}
);
}
});
});
/************
Validation
************/
// check date type
function IsDate(text) {
if ((new Date(text)) === null) return false;
if ((new Date(text)) == 'NaN') return false;
if ((new Date(text)) == 'Invalid Date') return false;
return true;
}
function IsNumber(str) {
return !Number.isNaN(parseInt(str));
// if (str.match(/[^0-9]+/)) {
// return false;
// }
// return true;
}
function IsDecimal(str) {
if (parseFloat(str)) {
return true;
} else {
return false;
}
}
function IsMail(str) {
if (!(str)) {
return true;
} else if (!str.match(/^[A-Za-z0-9]+[\w-\.-]+@[\w\.-]+\.\w{2,}$/)) {
return false;
}
return true;
}
/************
日付関係
************/
function ToDay() {
myDate = new Date();
theDate = myDate.getDate();
theFyear = myDate.getFullYear();
theYear = myDate.getYear(); theMonth = myDate.getMonth() + 1;
return theFyear + "/" + theMonth + "/" + theDate;
}
function addDate(date1Str, days) {
if (IsDate(date1Str)) {
var date1 = new Date(date1Str);
// パラメータで取得した日数を加算
date1.setDate(date1.getDate() + Number(days));
// Date型を(YYYY/MM/DD)形式へ成型して返却
return [date1.getFullYear(), date1.getMonth() + 1, date1.getDate()].join('/');
} else {
return date1Str;
}
}
function addMonth(date1Str, months) {
if (IsDate(date1Str)) {
var date1 = new Date(date1Str);
// パラメータで取得した月数を加算
var resultDate = new Date(date1.getTime());
resultDate.setMonth(date1.getMonth() + months);
// Date型を(YYYY/MM/DD)形式へ成型して返却
return [resultDate.getFullYear(), resultDate.getMonth() + 1, resultDate.getDate()].join('/');
} else {
return date1Str;
}
}
function DateInterval(date1, date2) {
if ((IsDate(date1)) && (IsDate(date2))) {
var d1 = new Date(date1);
var d2 = new Date(date2);
return Math.ceil((d2 - d1) / (60 * 60 * 24 * 1000));
} else {
return -1;
}
}
function GetBirthday(datevalue) {
var d1 = ConvertDate2(datevalue);
var d2 = ConvertDate2(ToDay());
if (d1) {
d1 = d1.replace(/\//g, "");
d2 = d2.replace(/\//g, "");
d1 = parseInt(d1);
d2 = parseInt(d2);
return parseInt((d2 - d1) / 10000);
}
return 0;
}
function DateString(date, format) {
if (IsDate(date)) {
date = new Date(date);
if (!format) format = 'yyyy-MM-dd hh:mm:ss.fs';
format = format.replace(/yyyy/g, date.getFullYear());
format = format.replace(/MM/g, ('0' + (date.getMonth() + 1)).slice(-2));
format = format.replace(/dd/g, ('0' + date.getDate()).slice(-2));
format = format.replace(/hh/g, ('0' + date.getHours()).slice(-2));
format = format.replace(/mm/g, ('0' + date.getMinutes()).slice(-2));
format = format.replace(/ss/g, ('0' + date.getSeconds()).slice(-2));
if (format.match(/fs/g)) {
var milliSeconds = ('00' + date.getMilliseconds()).slice(-3);
var length = format.match(/fs/g).length;
for (var i = 0; i < length; i++) format = format.replace(/S/, milliSeconds.substring(i, i + 1));
}
return format;
} else if ($.isNumeric(date)) {
try {
if (date.substr(8, 2) + date.substr(10, 2) + date.substr(12, 2)) {
date = date.substr(0, 4) + "/" + date.substr(4, 2) + "/" + date.substr(6, 2) + " " + date.substr(8, 2) + ":" + date.substr(10, 2) + ":" + date.substr(12, 2);
} else {
date = date.substr(0, 4) + "/" + date.substr(4, 2) + "/" + date.substr(6, 2);
}
return DateString(date, format);
} catch (e) {
return date;
}
} else {
return date;
}
}
/************
オブジェクト情報
************/
// Target Focus And Scroll
function ControlToFocus(ctl) {
if (ctl) {
//Focus
$(ctl).focus();
//Scroll
var t = parseInt(GetTop(ctl));
$(window).scrollTo(0, (t + 30));
if (t > 150) {
$(window).scrollTo(0, (t - 150));
} else {
$(window).scrollTo(0, 0);
}
}
}
// Get Control Position Left
function GetLeft(obj) {
return $(obj).offset().left;
}
// Get Control Position Top
function GetTop(obj) {
return $(obj).offset().top;
}
// Get Control Heigth
function GetHeight(obj) {
return $(obj).height();
}
// Get Control Width
function GetWidth(obj) {
return $(obj).width();
}
// Set Control Position Left
function SetLeft(obj, val) {
$(obj).offset({ top: GetTop(obj), left: val });
}
// Set Control Position Top
function SetTop(obj, val) {
$(obj).offset({ top: val, left: GetLeft(obj) });
}
// Set Control Heigth
function SetHeight(obj, val) {
$(obj).height(val);
}
// Set Control Width
function SetWidth(obj, val) {
$(obj).width(val);
}
// Set Position
function SetPosition(obj, left, top) {
$(obj).offset({ top: top, left: left });
}
function NumberCommaSplit(str) {
var num = new String(str).replace(/,/g, "");
while (num != (num = num.replace(/^(-?\d+)(\d{3})/, "$1,$2")));
return num;
}
/************
Loading
************/
function Loading_Start() {
$("#lock").show();
}
function Loading_Exit() {
$("#lock").hide();
}
/************
Popup Information
************/
function Pop_Save() {
$("#proccess_msg").html('保存完了
');
$("#proccess_msg").show();
setTimeout(function () { $("#proccess_msg").hide(); }, 3000);
}
function Pop_Remove() {
$("#proccess_msg").html('削除完了
');
$("#proccess_msg").show();
setTimeout(function () { $("#proccess_msg").hide(); }, 3000);
}
function Pop_Error(msg) {
$("#proccess_msg").html('' + ((msg) ? msg : "エラーが発生しています") + '
');
$("#proccess_msg").show();
setTimeout(function () { $("#proccess_msg").hide(); }, 3000);
}
function Pop_Message(msg) {
$("#proccess_msg").html('' + ((msg) ? msg : "お知らせ") + '
');
$("#proccess_msg").show();
setTimeout(function () { $("#proccess_msg").hide(); }, 3000);
}
/************
Browser Support
************/
function Browser() {
var ua = {};
ua.name = window.navigator.userAgent.toLowerCase();
ua.isIE = (ua.name.indexOf('msie') >= 0 || ua.name.indexOf('trident') >= 0);
ua.isiPhone = ua.name.indexOf('iphone') >= 0;
ua.isiPod = ua.name.indexOf('ipod') >= 0;
ua.isiPad = ua.name.indexOf('ipad') >= 0;
ua.isiOS = (ua.isiPhone || ua.isiPod || ua.isiPad);
ua.isAndroid = ua.name.indexOf('android') >= 0;
ua.isTablet = (ua.isiPad || (ua.isAndroid && ua.name.indexOf('mobile') < 0));
if (ua.isIE) {
ua.verArray = /(msie|rv:?)\s?([0-9]{1,})([\.0-9]{1,})/.exec(ua.name);
if (ua.verArray) {
ua.ver = parseInt(ua.verArray[2], 10);
}
}
if (ua.isiOS) {
ua.verArray = /(os)\s([0-9]{1,})([\_0-9]{1,})/.exec(ua.name);
if (ua.verArray) {
ua.ver = parseInt(ua.verArray[2], 10);
}
}
if (ua.isAndroid) {
ua.verArray = /(android)\s([0-9]{1,})([\.0-9]{1,})/.exec(ua.name);
if (ua.verArray) {
ua.ver = parseInt(ua.verArray[2], 10);
}
}
return ua;
}
/************
エレメントからの値操作
************/
function SetValue(ctl, val) {
var ret = '';
try {
//JQueryに変換
if (ctl.tagName) ctl = $(ctl);
//タグに応じた取得方法
if (ctl) {
switch (ctl[0].tagName.toUpperCase()) {
case "DIV":
case "SPAN":
if (ctl.find("input").length > 0) {
ctl.find("input").each(function () {
switch ($(this).attr("type").toUpperCase()) {
case "RADIO":
if ($(this).val() == val) {
$(this).prop("checked", true);
}
break;
case "CHECKBOX":
var vals = val.split(",");
$("input[name='" + ctl.attr("name") + "']").each(function () {
$(this).prop("checked", false);
for (i = 0; i < vals.length; i++) {
if ($(this).val() == vals[i]) {
$(this).prop("checked", true);
}
}
});
break;
default:
break;
}
});
} else {
ctl.html(val);
}
break;
case "A":
ctl.html(val);
break;
case "INPUT":
switch (ctl.attr("type").toUpperCase()) {
case "RADIO":
$("input[name='" + ctl.attr("name") + "']").each(function () {
if ($(this).val() == val) {
$(this).prop("checked", true);
} else {
$(this).prop("checked", false);
}
});
break;
case "CHECKBOX":
var vals = val.split(",");
$("input[name='" + ctl.attr("name") + "']").each(function () {
$(this).prop("checked", false);
for (i = 0; i < vals.length; i++) {
if ($(this).val() == vals[i]) {
$(this).prop("checked", true);
}
}
});
break;
default:
ctl.val(val);
break;
}
break;
case "TEXTAREA":
ctl.val(val);
break;
case "SELECT":
if (ctl.attr("class").indexOf("select2-offscreen") > -1) {
ctl.val(val);
ctl.select2();
} else {
ctl.val(val);
}
break;
}
}
} catch (e) {
alert(e);
}
return true;
}
function GetValue(ctl) {
var ret = '';
try {
//JQueryに変換
if (ctl.tagName) ctl = $(ctl);
//タグに応じた取得方法
if (ctl) {
switch (ctl[0].tagName.toUpperCase()) {
case "DIV":
case "SPAN":
if (ctl.find("input").length > 0) {
ctl.find("input").each(function () {
switch ($(this).attr("type").toUpperCase()) {
case "RADIO":
if ($(this).prop("checked")) {
ret = $(this).val();
}
break;
case "CHECKBOX":
if ($(this).prop("checked")) {
if (ret) ret += ",";
ret += $(this).val();
}
break;
default:
break;
}
});
} else {
ret = ctl.html();
}
break;
case "A":
ret = ctl.html();
break;
case "INPUT":
switch (ctl.attr("type").toUpperCase()) {
case "RADIO":
$("input[name='" + ctl.attr("name") + "']").each(function () {
if ($(this).prop("checked")) {
ret = $(this).val();
}
});
break;
case "CHECKBOX":
$("input[name='" + ctl.attr("name") + "']").each(function () {
if ($(this).prop("checked")) {
if (ret) ret += ",";
ret += $(this).val();
}
});
break;
case "DATE":
if (IsDate(ctl.val())) {
ret = DateString(ctl.val(), "yyyyMMdd");
}
break;
default:
ret = ctl.val();
break;
}
break;
case "TEXTAREA":
ret = ctl.val();
break;
case "SELECT":
ret = ctl.val();
break;
}
}
} catch (e) {
alert(e);
}
return ret;
}
function GetID(ctl) {
var ret = '';
try {
//JQueryに変換
if (ctl.tagName) ctl = $(ctl);
//タグに応じた取得方法
if (ctl) {
switch (ctl[0].tagName.toUpperCase()) {
case "DIV":
case "SPAN":
if (ctl.find("input").length > 0) {
ctl.find("input").each(function () {
switch ($(this).attr("type").toUpperCase()) {
case "RADIO":
ret = $(this).attr("name");
break;
case "CHECKBOX":
ret = $(this).attr("name");
break;
default:
break;
}
});
} else {
ret = (($(this).attr("id")) ? $(this).attr("id") : $(this).attr("name"));
}
break;
case "A":
ret = (($(this).attr("id")) ? $(this).attr("id") : $(this).attr("name"));
break;
case "INPUT":
switch (ctl.attr("type").toUpperCase()) {
case "RADIO":
$("input[name='" + ctl.attr("name") + "']").each(function () {
ret = $(this).attr("name");
});
break;
case "CHECKBOX":
$("input[name='" + ctl.attr("name") + "']").each(function () {
ret = $(this).attr("name");
});
break;
default:
ret = (($(this).attr("id")) ? $(this).attr("id") : $(this).attr("name"));
break;
}
break;
case "TEXTAREA":
ret = (($(this).attr("id")) ? $(this).attr("id") : $(this).attr("name"));
break;
case "SELECT":
ret = (($(this).attr("id")) ? $(this).attr("id") : $(this).attr("name"));
break;
}
}
} catch (e) {
alert(e);
}
return ((ret) ? ret : "");
}
/************************
** common.js [Write file text]
************************/
// use infomation
//
// html or aspx write example source in header tag [script type="text/javascript"]
//
// var ajax_top = /ajax
//
var q;
$(function () {
q = QueryStrings();
});
function QueryStrings() {
var vars = [], hash, qPos;
qPos = window.location.href.indexOf('?') + 1;
if (qPos > 0) {
var hashes = location.href.slice(qPos).split('&');
for (var i = 0; i < hashes.length; i++) {
hash = hashes[i].split('=');
vars.push(hash[0]);
var val = hash[1];
val = decodeURI(val);
vars[hash[0]] = val;
}
}
return vars;
}
function GetFormdata() {
var vars = new Object;
$("input, select, textarea").each(function () {
switch ($(this).attr("type").toUpperCase()) {
case "SUBMIT":
case "BUTTON":
case "IMAGE":
break;
case "RADIO":
if ($(this).prop("checked")) {
vars[$(this).attr("name")] = $(this).val();
}
break;
case "CHECKBOX":
if ($(this).prop("checked")) {
if (!vars[$(this).attr("name")]) {
vars[$(this).attr("name")] = $(this).val();
} else {
vars[$(this).attr("name")] += "," + $(this).val();
}
}
break;
default:
if ($(this).attr("name")) vars[$(this).attr("name")] = $(this).val();
}
});
return vars;
}
function set_radio(val, radio_name, defIdx) {
var flg = false;
for (i = 1; i < $("[name='" + radio_name + "']").length + 1; i++) {
if ($("#" + radio_name + "_" + String(i)).val() == val) {
$("#" + radio_name + "_" + String(i)).prop("checked", true);
flg = true;
} else {
$("#" + radio_name + "_" + String(i)).prop("checked", false);
}
}
if (!flg) {
if (defIdx) {
$("#" + radio_name + "_" + defIdx).prop("checked", true);
}
}
}
function get_radio(radio_name) {
for (i = 1; i < $("[name='" + radio_name + "']").length + 1; i++) {
if ($("#" + radio_name + "_" + String(i)).prop("checked")) {
return $("#" + radio_name + "_" + String(i)).val();
}
}
return "";
}
function ajax_error(XMLHttpRequest, textStatus, errorThrown) {
// , error: ajax_error
var msg = "【通信エラー】\n";
msg += "この画面をキャプチャーしてご連絡ください。\n";
msg += "XMLHttpRequest : " + XMLHttpRequest.status + "\n";
msg += "textStatus : " + textStatus + "\n";
msg += "errorThrown : " + errorThrown.message + "\n";
//alert(msg);
}
function ajax_complete(XMLHttpRequest, textStatus) {
// , complete: ajax_complete
var msg = "【Complete】\n";
msg += "XMLHttpRequest : " + XMLHttpRequest.status + "\n";
msg += "textStatus : " + textStatus + "\n";
alert(msg);
}
/**
ajax call (base)
**/
function ajax_proc(ashx, parms, async, success, error) {
if (async) async = true;
else async = false;
if (ashx) {
$.ajax({
url: ashx
, type: 'POST'
, cache: false
, dataType: 'json'
, processData: false
, contentType: false
, async: async
, data: parms
, success: function (res) {
if (res["result"] == "success") {
if (success) success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[ashx]を指定してください。");
}
}
/**
ajax util
**/
function get_youtube(youtubeId, success, error) {
$.ajax({
url: ajax_top + '/youtube.ashx'
, type: 'POST'
, cache: false
, async: false
, dataType: 'json'
, data: {
uid: youtubeId
}
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
}
function ajax_set_address(obj1, obj2, success, error) {
if ((obj1) && (obj2)) {
obj1.keyup(function () { ajax_get_address(obj1.val() + obj2.val(), success, error); });
obj2.keyup(function () { ajax_get_address(obj1.val() + obj2.val(), success, error); });
} else if (obj1) {
obj1.keyup(function () { ajax_get_address(obj1.val(), success, error); });
} else if (obj2) {
obj2.keyup(function () { ajax_get_address(obj2.val(), success, error); });
}
}
function ajax_get_address(val, success, error) {
if (val.length == 7) {
$.ajax({
url: "https://ajax.deprog.jp/post_get.ashx"
, type: 'POST'
, cache: false
, dataType: 'json'
, data: {
postno: val
}
, success: function (res) {
if (res["result"] == "success") {
if (success) success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
}
}
function ajax_requestinfo(async, success, error) {
if (async) async = true;
else async = false;
$.ajax({
url: ajax_top + '/requestinfo.ashx'
, type: 'POST'
, cache: false
, async: async
, dataType: 'json'
, success: function (res) {
if (res["result"] == "success") {
if (success) success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
}
function ajax_decrypt(val, success, error) {
$.ajax({
url: ajax_top + '/sec_decrypt.ashx'
, type: 'POST'
, cache: false
, async: false
, dataType: 'json'
, data: {
val: val
}
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
}
function ajax_encrypt(val, success, error) {
$.ajax({
url: ajax_top + '/sec_encrypt.ashx'
, type: 'POST'
, cache: false
, async: false
, dataType: 'json'
, data: {
val: val
}
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
}
function ajax_mail(mt, ms, mb, success, error) {
$.ajax({
url: ajax_top + '/mail.ashx'
, type: 'POST'
, cache: false
, async: false
, dataType: 'json'
, data: {
to: mt
, subject: ms
, body: mb
}
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
}
function ajax_ekidata(prefObj, lineObj, stationObj, stationObj2) {
//initialize
if (prefObj) {
if (prefObj.children().length == 0) {
$.ajax({
url: ajax_top + '/ekidata.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: true
, success: function (res) {
if (res["result"] == "success") {
prefObj.children().remove();
prefObj.append($("').val("").html("選択してください"));
obj.append($('').val("北海道").html("北海道"));
obj.append($('').val("青森県").html("青森県"));
obj.append($('').val("岩手県").html("岩手県"));
obj.append($('').val("宮城県").html("宮城県"));
obj.append($('').val("秋田県").html("秋田県"));
obj.append($('').val("山形県").html("山形県"));
obj.append($('').val("福島県").html("福島県"));
obj.append($('').val("茨城県").html("茨城県"));
obj.append($('').val("栃木県").html("栃木県"));
obj.append($('').val("群馬県").html("群馬県"));
obj.append($('').val("埼玉県").html("埼玉県"));
obj.append($('').val("千葉県").html("千葉県"));
obj.append($('').val("東京都").html("東京都"));
obj.append($('').val("神奈川県").html("神奈川県"));
obj.append($('').val("山梨県").html("山梨県"));
obj.append($('').val("長野県").html("長野県"));
obj.append($('').val("新潟県").html("新潟県"));
obj.append($('').val("富山県").html("富山県"));
obj.append($('').val("石川県").html("石川県"));
obj.append($('').val("福井県").html("福井県"));
obj.append($('').val("岐阜県").html("岐阜県"));
obj.append($('').val("静岡県").html("静岡県"));
obj.append($('').val("愛知県").html("愛知県"));
obj.append($('').val("三重県").html("三重県"));
obj.append($('').val("滋賀県").html("滋賀県"));
obj.append($('').val("京都府").html("京都府"));
obj.append($('').val("大阪府").html("大阪府"));
obj.append($('').val("兵庫県").html("兵庫県"));
obj.append($('').val("奈良県").html("奈良県"));
obj.append($('').val("和歌山県").html("和歌山県"));
obj.append($('').val("鳥取県").html("鳥取県"));
obj.append($('').val("島根県").html("島根県"));
obj.append($('').val("岡山県").html("岡山県"));
obj.append($('').val("広島県").html("広島県"));
obj.append($('').val("山口県").html("山口県"));
obj.append($('').val("徳島県").html("徳島県"));
obj.append($('').val("香川県").html("香川県"));
obj.append($('').val("愛媛県").html("愛媛県"));
obj.append($('').val("高知県").html("高知県"));
obj.append($('').val("福岡県").html("福岡県"));
obj.append($('').val("佐賀県").html("佐賀県"));
obj.append($('').val("長崎県").html("長崎県"));
obj.append($('').val("熊本県").html("熊本県"));
obj.append($('').val("大分県").html("大分県"));
obj.append($('').val("宮崎県").html("宮崎県"));
obj.append($('').val("鹿児島県").html("鹿児島県"));
obj.append($('').val("沖縄県").html("沖縄県"));
obj.val(val);
}
ajax_top = "_deprog\ajax"; // REWRITE
/************************
** common.db.js [Write file text]
************************/
/**
Deprog Tool Kit 2015/12/01
PartsManager.DataManager
PartsManager.DataManagerSP
PartsManager.MastaManager
**/
function ajax_get(extension, datakey, uid, arrCols) {
// require parms
var parms = new Object;
parms["datakey"] = datakey;
parms["extension"] = extension;
//use columns
if (arrCols) {
for (i = 0; i < arrCols.length; i++) {
parms["Col_" + String(i)] = arrCols[i];
}
}
//one data
parms["sCol_0"] = "ID";
parms["sVal_0"] = uid;
var ret = new Object;
$.ajax({
url: ajax_top + '/list.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: false
, data: parms
, success: function (res) {
if (res["result"] == "success") {
if (res["data"].length > 0) {
ret = res["data"][0];
} else {
ret = null;
}
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
return ret;
}
function ajax_list(extension, datakey, arrCols, htCols, sort, async, success, error, isOne) {
if (async) async = true;
else async = false;
if (arrCols) {
// require parms
var parms = new Object;
parms["datakey"] = datakey;
parms["extension"] = extension;
//check parms
for (i = 0; i < arrCols.length; i++) {
parms["Col_" + String(i)] = arrCols[i];
}
//search column
if (htCols) {
var idx = 0;
for (var key in htCols) {
parms["sCol_" + String(idx)] = key;
parms["sVal_" + String(idx)] = htCols[key];
idx++;
}
}
//sort
if (sort) parms["sort"] = sort;
//isOne
if (isOne) parms["isOne"] = "1";
if (success) {
$.ajax({
url: ajax_top + '/list.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
} else {
alert("引数[arrCols]を指定してください。");
}
}
function ajax_list2(extension, datakey, sqlName, htCols, sort, async, success, error) {
if (async) async = true;
else async = false;
// require parms
var parms = new Object;
parms["datakey"] = datakey;
parms["extension"] = extension;
parms["sqlName"] = sqlName;
//search column
if (htCols) {
var idx = 0;
for (var key in htCols) {
parms["sCol_" + String(idx)] = key;
parms["sVal_" + String(idx)] = htCols[key];
idx++;
}
}
//sort
if (sort) parms["sort"] = sort;
if (success) {
$.ajax({
url: ajax_top + '/list.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
}
function ajax_list_sql(extension, datakey, sql, async, success) {
if (async) async = true;
else async = false;
// require parms
var parms = new Object;
parms["datakey"] = datakey;
parms["extension"] = extension;
parms["sql"] = sql;
if (success) {
$.ajax({
url: ajax_top + '/list_sql.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: ajax_error
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
}
function ajax_select(targetID, extension, datakey, parms, links, sDom, iDisplayLength, aaSorting, fnCreatedRow, fnDrawCallback, sqlName, scrollY, scrollHeight) {
//validation
var msg = new Array;
if (!targetID) msg.push("引数[targetID]を指定してください。");
if ($('#' + targetID).length == 0) msg.push("指定したテーブルが存在しません。");
if (!extension) msg.push("引数[extension]を指定してください。");
if (!datakey) msg.push("引数[datakey]を指定してください。");
if (!parms) msg.push("引数[parms]を指定してください。");
if (!sDom) sDom = '<"H"lfip>t<"F"ip>';
if (!iDisplayLength) iDisplayLength = 25;
if (msg.length > 0) {
alert(msg.join("\n"));
return false;
}
//default
for (i = 0; i < parms.length; i++) {
if (!parms[i]["title"]) parms[i]["title"] = "COL_" + String(i);
if (!parms[i]["width"]) parms[i]["width"] = "";
if (!parms[i]["sCol"]) parms[i]["sCol"] = "";
if (!parms[i]["sType"]) parms[i]["sType"] = "";
if (!parms[i]["sInit"]) {
if (parms[i]["sInit"] == "0") {
//ZERO
} else {
parms[i]["sInit"] = "";
}
}
if (!parms[i]["sWhere"]) parms[i]["sWhere"] = "";
if (!parms[i]["sBeetween"]) parms[i]["sBeetween"] = "";
if (!parms[i]["sOr"]) parms[i]["sOr"] = "";
else parms[i]["sOr"] = "1";
if (!parms[i]["sSort"]) parms[i]["sSort"] = "";
if (!parms[i]["bSortable"]) parms[i]["bSortable"] = false;
if (!parms[i]["bVisible"]) parms[i]["bVisible"] = false;
if (!parms[i]["search"]) parms[i]["search"] = false;
if (!parms[i]["add"]) parms[i]["add"] = false;
// JOIN 20210816
if (!parms[i]["join"]) parms[i]["join"] = "";
}
//table clear
if (targetID) $("#" + targetID).html("");
//table options
var isSearch = false;
var isAdd = false;
for (i = 0; i < parms.length; i++) {
if (parms[i]["search"]) isSearch = true;
if (parms[i]["add"]) isAdd = true;
}
//make title
var thead_title = $("
");
var tfoot_title = $("
");
var thead_2 = $("
");
var thead_3 = $("
");
for (i = 0; i < parms.length; i++) {
//input type
var inputType = "text";
switch (parms[i]["sType"]) {
case "int":
case "numeric":
parms[i]["sType"] = "numeric";
inputType = "number";
break;
case "date":
parms[i]["sType"] = "";
inputType = "date";
break;
case "string":
default:
parms[i]["sType"] = "";
break;
}
//title
if ((!parms[i]["width"]) || (parms[i]["width"] == "auto") || (!parms[i]["bVisible"])) {
thead_title.append('' + parms[i]["title"] + ' | ');
tfoot_title.append('' + parms[i]["title"] + ' | ');
} else {
thead_title.append('' + parms[i]["title"] + ' | ');
tfoot_title.append('' + parms[i]["title"] + ' | ');
}
//search
if (isSearch) {
if (parms[i]["sCol"]) {
switch (parms[i]["search"]) {
case "auto":
case true:
thead_2.append(' | ');
break;
default:
if (parms[i]["sCol"] != "DUMMY") {
thead_2.append(' | ');
} else {
thead_2.append(' | ');
}
break;
}
} else {
thead_2.append(' | ');
}
}
//add
if (isAdd) {
if (parms[i]["sCol"]) {
switch (parms[i]["add"]) {
case "auto":
case true:
thead_3.append(' | ');
break;
default:
if (parms[i]["sCol"] != "DUMMY") {
thead_3.append(' | ');
} else {
thead_3.append(' | ');
}
break;
}
} else {
thead_3.append(' | ');
}
}
}
//bulid table layout
var thead = $("").append(thead_title);
var tbody = $("").append('データ受信中... |
');
var tfoot = $("").append(tfoot_title);
if (isSearch) tfoot.append(thead_2);
if (isAdd) tfoot.append(thead_3);
//paging off 20220524
if ($('#' + targetID).data("pagingoffH") != "1") $('#' + targetID).append(thead);
$('#' + targetID).append(tbody);
if ($('#' + targetID).data("pagingoffF") != "1") $('#' + targetID).append(tfoot);
//$('#' + targetID).append(thead).append(tbody).append(tfoot);
//ajax path and post data
var ajax_data = new Object;
var ajax_path = ajax_top + '/select.ashx';
if (sqlName) {
ajax_path = ajax_top + '/select_manual.ashx';
ajax_data['sqlName'] = sqlName;
}
ajax_data['extension'] = extension;
ajax_data['datakey'] = datakey;
for (i = 0; i < parms.length; i++) {
ajax_data["sCol_" + String(i)] = parms[i]["sCol"];
ajax_data["sType_" + String(i)] = parms[i]["sType"];
ajax_data["sInit_" + String(i)] = parms[i]["sInit"];
ajax_data["sWhere_" + String(i)] = parms[i]["sWhere"];
ajax_data["sBeetween_" + String(i)] = parms[i]["sBeetween"];
ajax_data["sOr_" + String(i)] = parms[i]["sOr"];
ajax_data["sSort_" + String(i)] = parms[i]["sSort"];
// JOIN 20210816
ajax_data["join_" + String(i)] = parms[i]["join"];
// JOIN 20210816
}
if (links) {
for (i = 0; i < links.length; i++) {
ajax_data["lnkName_" + String(i)] = links[i]["name"];
ajax_data["lnkCol_" + String(i)] = links[i]["col"];
ajax_data["lnkFrom_" + String(i)] = links[i]["from"];
ajax_data["lnkFromCol_" + String(i)] = links[i]["from-col"];
}
}
//aaSorting
if (aaSorting) {
for (i = 0; i < aaSorting.length; i++) {
if ($.isNumeric(aaSorting[i][0])) {
continue;
} else {
for (j = 0; j < parms.length; j++) {
if (aaSorting[i][0] == parms[j]["sCol"]) {
aaSorting[i][0] = j;
break;
}
}
}
}
}
//aoColumns
var aoColumns = new Array;
for (i = 0; i < parms.length; i++) {
if (parms[i]["sType"] == "numeric") {
aoColumns.push({ sType: parms[i]["sType"], bSortable: parms[i]["bSortable"], bVisible: parms[i]["bVisible"] });
} else {
aoColumns.push({ bSortable: parms[i]["bSortable"], bVisible: parms[i]["bVisible"] });
}
}
//bulid datatables
var oTable;
$('#' + targetID).removeClass("tableUI");
oTable = $('#' + targetID).DataTable({
"sDom": sDom
, "buttons": ['excel', 'pdf', 'print']
, "bAutoWidth": false
, "iDisplayLength": iDisplayLength
, "aaSorting": aaSorting
, "aoColumns": aoColumns
// use ajax
, "bProcessing": true
, "bDeferRender": true
, "bServerSide": true
, "ajax": {
url: ajax_path
, type: 'POST'
, dataType: "json"
, cache: false
, data: ajax_data
, dataSrc: function (json) {
if (json["custom-error"] == "1") {
alert("[DataTable]\n" + targetID + "\n[システム障害]\n" + json["custom-error-message"] + "\n[指定した列情報]\n" + json["custom-error-columns"]);
return true;
} else {
// var val = new Array;
// for (var key in json) {
// val.push("["+key+"]"+json[key]);
// }
return json["aaData"];
}
}
}
// make row
, "fnCreatedRow": function (nRow, aData, iDataIndex) {
var ret = new Object;
for (i = 0; i < parms.length; i++) {
ret[parms[i]["sCol"].replace(".", "_")] = aData[i];
ret[i] = aData[i];
}
aData = ret;
fnCreatedRow(nRow, aData, iDataIndex, targetID);
}
// complete
, "fnDrawCallback": function (oSettings) {
$(".datatables-top td, .datatables-new td").removeClass("ui-state-default");
if (scrollY) $(window).trigger("resize");
$(".dataTables_filter").hide();
$(".dataTables_empty").width($('#' + targetID).width());
fnDrawCallback(oSettings, targetID);
}
});
$('#' + targetID + " .datatables-top").appendTo($('#' + targetID + " thead"));
$('#' + targetID + " .datatables-new").appendTo($('#' + targetID + " thead"));
// if (!scrollY) {
// $('#' + targetID + " tfoot").remove();
// }
//event
if (scrollY) {
$(window).resize(function () {
windows_table_fix(targetID, scrollHeight);
});
$("#" + targetID + " tbody").scroll(table_height);
}
//base search
for (i = 0; i < parms.length; i++) {
if (parms[i]["sInit"]) {
SearchColSetting(oTable, i, parms[i]["sInit"]);
} else if (parms[i]["sInit"] == "0") {
SearchColSetting(oTable, i, parms[i]["sInit"]);
}
}
//search columns
for (i = 0; i < parms.length; i++) {
//search
if (isSearch) {
if (parms[i]["sCol"]) {
if (parms[i]["sCol"] != "DUMMY") {
switch (parms[i]["search"]) {
case "auto":
case true:
$('#' + targetID + '_' + parms[i]["sCol"].replace(".", "_") + '_search input').keyup(function (e) {
if (e.keyCode == 13) {
// oTable.fnFilter($(this).val(), $(this).attr("column_index"));
if ($(this).attr("column_index")) {
SearchColSetting(oTable, $(this).attr("column_index"), $(this).val());
}
oTable.draw();
}
});
break;
default:
break;
}
}
}
}
}
//complete
return oTable;
}
// column
// function SearchColSetting(oSettings, column_index, value) {
// if (oSettings) {
// for (iCol = 0; iCol < oSettings.aoPreSearchCols.length; iCol++) {
// switch (iCol) {
// case column_index:
// oSettings.aoPreSearchCols[iCol].sSearch = value;
// break;
// }
// }
// }
// }
function SearchColSetting(otable, column_index, value) {
if (otable) {
if (otable.column(column_index)) {
otable.column(column_index).search(value);
}
}
}
function windows_table_fix(targetID, scrollHeight) {
var b = Browser();
var cntTh = $("#" + targetID + " thead tr:eq(0) th").length;
for (i = 0; i < cntTh; i++) {
var wTh = parseInt($("#" + targetID + " thead tr:first th:eq(" + String(i) + ")").innerWidth());
if (i < (cntTh - 1)) {
$("#" + targetID + " tbody tr:first td:eq(" + String(i) + ")").width(wTh - 19);
} else {
if (b.isIE) {
$("#" + targetID + " tbody tr:first td:eq(" + String(i) + ")").width(wTh - 37);
} else {
$("#" + targetID + " tbody tr:first td:eq(" + String(i) + ")").width(wTh - 38);
}
}
}
// width
var now_width = parseInt($("#" + targetID).outerWidth());
// height
if (scrollHeight) {
$("#" + targetID + " tbody").css({ display: "block", maxHeight: String(scrollHeight) + "px", overflowY: "scroll" }).width(now_width - 3);
} else {
$("#" + targetID + " tbody").css({ display: "block", maxHeight: "100px", overflowY: "scroll" }).width(now_width - 3);
}
}
function table_height(e) {
var p = parseInt($(this).scrollTop());
if (p > 0) {
if ($(".auto_scroll_hidden").is(":hidden")) {
//ok
} else {
$(".auto_scroll_hidden").slideUp("fast");
$(window).trigger("resize");
}
} else {
if ($(".auto_scroll_hidden").is(":hidden")) {
$(".auto_scroll_hidden").slideDown("fast");
$(window).trigger("resize");
} else {
//ok
}
}
//alert(p);
}
function ajax_update(extension, datakey, uid, htCols, async, success, error) {
if (async) async = true;
else async = false;
if (htCols) {
// require parms
var parms = new Object;
parms["uid"] = uid;
parms["datakey"] = datakey;
parms["extension"] = extension;
//check column
if (htCols) {
var idx = 0;
for (var key in htCols) {
parms["Col_" + String(idx)] = key;
parms["Val_" + String(idx)] = htCols[key];
idx++;
}
}
if (success) {
$.ajax({
url: ajax_top + '/update.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
} else {
alert("引数[htCols]を指定してください。");
}
}
function ajax_copy(extension, datakey, uid, async, success, error) {
var arrCols = new Array, htCols = new Object;
// column list
for (i = 1; i < 5; i++) {
arrCols.push("KEY" + String(i));
}
for (i = 1; i < 100; i++) {
arrCols.push("PARM" + String(i));
}
// where
htCols["ID"] = uid;
// data load
ajax_list(extension, datakey, arrCols, htCols, "", true, function (res) {
if (res["data"].length > 0) {
// copy main
htCols = new Object;
for (var key in res["data"][0]) {
htCols[key] = res["data"][0][key];
}
ajax_update(extension, datakey, "", htCols, async, success, error);
} else {
alert("指定した元データを取得できませんでした。");
}
});
}
function ajax_remove(extension, datakey, uid, async, success, error) {
if (async) async = true;
else async = false;
var parms = new Object;
parms["uid"] = uid;
parms["datakey"] = datakey;
parms["extension"] = extension;
if (success) {
$.ajax({
url: ajax_top + '/remove.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] == "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
}
function ajax_fileupload(targetId, extension, datakey, uid, idx, acceptedFiles, parallelUploads, maxFiles, success) {
if (success) {
if (!parallelUploads) parallelUploads = 1;
if (!maxFiles) maxFiles = 1;
var ajax_path = ajax_top + '/fileupload.ashx';
ajax_path += "?extension=" + extension;
ajax_path += "&datakey=" + datakey;
ajax_path += "&uid=" + uid;
ajax_path += "&idx=" + idx;
// destroy
try { Dropzone.forElement("#" + targetId).destroy(); } catch (e) { }
$("#" + targetId).dropzone({
url: ajax_path
, parallelUploads: parallelUploads
, maxFiles: maxFiles
, acceptedFiles: acceptedFiles
, success: function (_file, _return, _xml) {
_file.previewElement.classList.add("dz-success");
// _return["pid"]
// _return["src"]
success(_file, _return, _xml);
}
, complete: function () {
setTimeout(function () {
$(".dz-preview:eq(0)").remove();
if ($(".dz-preview").length == 0) {
$(".dropzone").removeClass("dz-started");
}
}, 1000);
}
, init: function () {
Dropzone.autoDiscover = false;
Dropzone.myAwesomeDropzone = false;
}
, error: function (_file, _error_msg) {
alert(_error_msg);
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
}
function ajax_fileupload_key1(targetId, extension, datakey, key1, idx, acceptedFiles, parallelUploads, maxFiles, success) {
if (success) {
if (!parallelUploads) parallelUploads = 1;
if (!maxFiles) maxFiles = 1;
var ajax_path = ajax_top + '/fileupload.ashx';
ajax_path += "?extension=" + extension;
ajax_path += "&datakey=" + datakey;
ajax_path += "&key1=" + key1;
ajax_path += "&idx=" + idx;
// destroy
try { Dropzone.forElement("#" + targetId).destroy(); } catch (e) { }
$("#" + targetId).dropzone({
url: ajax_path
, parallelUploads: parallelUploads
, maxFiles: maxFiles
, acceptedFiles: acceptedFiles
, success: function (_file, _return, _xml) {
_file.previewElement.classList.add("dz-success");
// _return["pid"]
// _return["src"]
success(_file, _return, _xml);
}
, complete: function () {
setTimeout(function () {
$(".dz-preview:eq(0)").remove();
if ($(".dz-preview").length == 0) {
$(".dropzone").removeClass("dz-started");
}
}, 1000);
}
, init: function () {
Dropzone.autoDiscover = false;
Dropzone.myAwesomeDropzone = false;
}
, error: function (_file, _error_msg) {
alert(_error_msg);
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
}
function ajax_fileupload_manual(targetId, extension, datakey, uid, idx, key1, async, success) {
if (success) {
if (async) async = true;
else async = false;
var parms = new FormData();
parms.append("datakey", datakey);
parms.append("extension", extension);
parms.append("uid", uid);
parms.append("idx", idx);
parms.append("upfile", $("#" + targetId).prop("files")[0]);
var ajax_path = ajax_top + '/fileupload_manual.ashx';
ajax_proc(ajax_path, parms, async, function (res) { success(res); });
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
}
function ajax_download(real, name) {
//proc start
var url = ajax_top + "/download.aspx?real=" + real + "&name=" + name;
if (Browser().isIE) url += "&enc=shift-jis";
$("#download").attr("src", "");
$("#download").attr("src", url);
}
function ajax_autocomplete(className, extension, datakey, column, async, selected, opened, success, error) {
if (async) async = true;
else async = false;
// require parms
var parms = new Object;
parms["datakey"] = datakey;
parms["extension"] = extension;
parms["column"] = column;
$.ajax({
url: ajax_top + '/autocomplete.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] == "success") {
if (success) {
success(res);
} else {
$("." + className).autocomplete({
source: res["data"]
, select: ((selected) ? selected : null)
, open: ((opened) ? opened : null)
});
}
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
}
/************
DeprogToolkit Suport
************/
// submit control is lock on release
$(function () {
// //file
// $FileUI();
// //file
// $FileUI2();
// //ckeditor
// $CKEditorUI();
// // CKEDITOR.instances['Element ID'].getData()
// // CKEDITOR.instances['Element ID'].setData()
// //datatables
// $TableUI();
// //AutoSave
// $FormUI();
});
function $FileUI(targetId) {
$(".fileUI").each(function () {
if (targetId) {
if ($(this).attr("id") == targetId) {
//OK
} else {
return;
}
}
var runDestroyProc = false;
try { runDestroyProc = true; Dropzone.forElement($(this).get(0)).destroy(); } catch (e) { }
$(this).html("");
var msg = new Array;
if (!$(this).data("uid")) msg.push("FILE UI[uid]を指定してください");
if (!$(this).data("extension")) msg.push("FILE UI[data-extension]を指定してください");
if (!$(this).data("name")) msg.push("FILE UI[data-name]を指定してください");
if (!$(this).data("index")) msg.push("FILE UI[data-index]を指定してください");
// if (!$(this).data("type")) msg.push("FILE UI[data-type]を指定してください");
if (msg.length > 0) {
alert(msg.join("\n"));
$(this).css("border", "10px solid #f00");
return;
}
var message = $('クリック or ドラッグ
' + (($(this).data("type")) ? '[' + $(this).data("type").replace(",", "") + ']' : '') + '');
$(this).append(message);
if (!$(this).hasClass("dropzone")) $(this).addClass("dropzone");
var ajax_path = ajax_top + '/fileupload.ashx'
+ "?uid=" + $(this).data("uid")
+ "&extension=" + $(this).data("extension")
+ "&datakey=" + $(this).data("name")
+ "&idx=" + $(this).data("index");
$(this).dropzone({
url: ajax_path
, parallelUploads: 1
, maxFiles: 100
, acceptedFiles: (($(this).data("type")) ? $(this).data("type") : "")
, addRemoveLinks: false
, success: function (_file, _return, _xml) {
var el = $(_file.previewElement);
_file.previewElement.classList.add("dz-success");
$(_file.previewElement).attr("pid", _return["pid"]);
}
, complete: function (_file) {
var el = $(this.element);
setTimeout(function () {
if (el.find(".dz-preview").length == 1) {
$(".dz-image").remove();
$(".dz-size").remove();
$(".dz-progress").remove();
}
}, 1000);
}
, sending: function (_file) {
var el = $(this.element);
if (el.find(".dz-preview").length > 1) {
var posistion = el.find(".dz-preview").length - 1;
el.find(".dz-preview:eq(" + String(posistion) + ")").remove();
}
}
, init: function () {
Dropzone.autoDiscover = false;
Dropzone.myAwesomeDropzone = false;
var el = $(this.element);
ajax_list(el.data("extension"), el.data("name"), ["DATE2", "URL" + el.data("index"), "FUP" + el.data("index")], { ID: el.data("uid") }, "", false, function (res) {
if (res["data"].length == 0) return;
if (!res["data"][0]["URL" + el.data("index")]) return;
var html = '';
html += '\n';
html += '
\n';
if (res["data"][0]["FUP" + el.data("index")].toLowerCase().indexOf(".pdf") >= 0) {
//PDF
html += '
' + res["data"][0]["FUP" + el.data("index")] + '
\n';
html += '
ダウンロード\n';
} else {
//Image
html += '

\n';
}
html += '
\n';
html += '
削除\n';
html += '
\n';
el.append(html);
el.find(".dz-remove").click(function () {
if (confirm("ファイルを削除しても良いですか?")) {
var delUid = $(this).data("uid");
var delIdx = $(this).data("index");
var ajax_path = ajax_top + '/fileupload.ashx'
+ "?uid=" + delUid
+ "&extension=" + $(this).data("extension")
+ "&datakey=" + $(this).data("name")
+ "&idx=" + delIdx
+ "&del=1";
ajax_proc(ajax_path, null, false, function () {
$(".fileUI").each(function () { if (($(this).data('uid') == delUid) && ($(this).data('index') == delIdx)) $(this).find('.dz-preview').remove(); });
//$(".fileUI[data-uid='" + delUid + "'][data-index='" + delIdx + "'] .dz-preview").remove();
});
}
});
});
}
, error: function (_file, _error_msg) {
var el = $(this.element);
alert(_error_msg);
}
, removedfile: function (_file) {
if (runDestroyProc) return true;
var el = $(this.element);
if (confirm("ファイルを削除しても良いですか?")) {
var ajax_path = ajax_top + '/fileupload.ashx'
+ "?uid=" + el.data("uid")
+ "&extension=" + el.data("extension")
+ "&datakey=" + el.data("name")
+ "&idx=" + el.data("index")
+ "&del=1";
ajax_proc(ajax_path, null, false, function () {
el.find(".dz-preview").remove();
});
}
}
, dictInvalidFileType: "アップロードできるファイルを選択してください。[" + $(this).data("type") + "]"
, dictRemoveFile: "削除"
});
});
}
function $FileUI2(targetId) {
$(".fileUI2").each(function () {
if (targetId) {
if ($(this).attr("id") == targetId) {
//OK
} else {
return;
}
}
var runDestroyProc = false;
try { runDestroyProc = true; Dropzone.forElement($(this).get(0)).destroy(); } catch (e) { }
$(this).html("");
runDestroyProc = false;
var msg = new Array;
if (!$(this).data("uid")) msg.push("FILE UI 2[uid]を指定してください");
if (!$(this).data("extension")) msg.push("FILE UI 2[data-extension]を指定してください");
if (!$(this).data("name")) msg.push("FILE UI 2[data-name]を指定してください");
//if (!$(this).data("type")) msg.push("FILE UI 2[type]を指定してください");
if (msg.length > 0) {
alert(msg.join("\n"));
$(this).css("border", "10px solid #f00");
return;
}
var message = $('クリック or ドラッグ
' + (($(this).data("type")) ? '[' + $(this).data("type").replace(",", "") + ']' : '') + '');
$(this).append(message);
if (!$(this).hasClass("dropzone")) $(this).addClass("dropzone");
var ajax_path = ajax_top + '/fileupload.ashx'
+ "?key1=" + $(this).data("uid")
+ "&extension=" + $(this).data("extension")
+ "&datakey=" + $(this).data("name");
$(this).dropzone({
url: ajax_path
, parallelUploads: 1
, maxFiles: 100
, acceptedFiles: (($(this).data("type")) ? $(this).data("type") : "")
, addRemoveLinks: true
, success: function (_file, _return, _xml) {
var el = $(this.element);
_file.previewElement.classList.add("dz-success");
$(_file.previewElement).attr("pid", _return["pid"]);
}
, complete: function (_file) {
var el = $(this.element);
}
, sending: function (_file) {
var el = $(this.element);
if (el.find(".dz-preview").length > 1) {
var posistion = el.find(".dz-preview").length - 1;
el.find(".dz-preview:eq(" + String(posistion) + ")").remove();
}
if (el.find(".dz-preview .dz-progress").length == 0) {
setTimeout(function () { $FileUI2(el.attr("id")); }, 1000);
}
}
, init: function () {
Dropzone.autoDiscover = false;
Dropzone.myAwesomeDropzone = false;
var el = $(this.element);
var htWhere = new Object;
htWhere["KEY1"] = el.data("uid");
if (el.data("key2")) htWhere["KEY2"] = el.data("key2");
if (el.data("key3")) htWhere["KEY3"] = el.data("key3");
if (el.data("key4")) htWhere["KEY4"] = el.data("key4");
if (el.data("key5")) htWhere["KEY5"] = el.data("key5");
ajax_list(el.data("extension"), el.data("name"), ["ID", "URL1", "FUP1"], htWhere, "", false, function (res) {
if (res["data"].length == 0) return;
for (i = 0; i < res["data"].length; i++) {
var html = '';
html += '\n';
html += '
\n';
if (res["data"][0]["FUP1"].toLowerCase().indexOf(".pdf") >= 0) {
//PDF
html += '
' + res["data"][i]["FUP1"] + '
\n';
html += '
ダウンロード\n';
} else {
//Image
html += '

\n';
}
html += '
\n';
html += '
削除\n';
html += '
\n';
el.append(html);
}
el.find(".dz-remove").click(function () {
if (confirm("ファイルを削除しても良いですか?")) {
var delUid = $(this).data("uid");
var ajax_path = ajax_top + '/fileupload.ashx'
+ "?uid=" + delUid
+ "&key1=" + $(this).data("key1")
+ "&extension=" + $(this).data("extension")
+ "&datakey=" + $(this).data("name")
+ "&del=1";
ajax_proc(ajax_path, null, false, function () {
$(".fileUI2 .dz-preview[pid='" + delUid + "']").remove();
});
//alert(el.data("uid"));
}
});
});
}
, error: function (_file, _error_msg) {
var el = $(this.element);
alert(_error_msg);
}
, canceled: function (_file) {
if (runDestroyProc) return true;
}
, removedfile: function (_file) {
if (runDestroyProc) return true;
// var el = $(this.element);
// if (confirm("ファイルを削除しても良いですか?")) {
// var ajax_path = ajax_top + '/fileupload.ashx'
// + "?uid=" + $(_file.previewElement).attr("pid")
// + "&key1=1"
// + "&extension=" + el.data("extension")
// + "&datakey=" + el.data("name")
// + "&del=1";
// ajax_proc(ajax_path, null, false, function () {
// $(_file.previewElement).remove();
// });
// }
}
, dictInvalidFileType: "アップロードできるファイルを選択してください。[" + $(this).data("type") + "]"
});
});
}
var isStatus_FileUI3 = false;
function $FileUI3() {
$(".fileUI3").each(function () {
var runDestroyProc = false;
try { runDestroyProc = true; Dropzone.forElement($(this).get(0)).destroy(); } catch (e) { }
var msg = new Array;
if (!$(this).data("handler")) msg.push("FILE UI 3[handler]を指定してください");
if (msg.length > 0) {
alert(msg.join("\n"));
$(this).css("border", "10px solid #f00");
return;
}
if (!$(this).hasClass("dropzone")) $(this).addClass("dropzone");
var ajax_path = $(this).data("handler");
var callbackFileUI3 = $(this).data("callback");
$(this).dropzone({
url: ajax_path
, parallelUploads: 1
, maxFiles: 100
, acceptedFiles: (($(this).data("type")) ? $(this).data("type") : "")
, addRemoveLinks: true
, success: function (_file, _return, _xml) {
var el = $(this.element);
if (isStatus_FileUI3) {
$("#lock").hide();
if (_return["result"] == "success") {
// $("#debug").html("");
// for (var key in _return) {
// $("#debug").append('' + "[" + key + "]" + _return[key] + '
');
// }
// $("#debug").show();
if (callbackFileUI3) {
callbackFileUI3 += "(el, _return)";
eval(callbackFileUI3);
return;
}
} else {
alert(_return["msg"]);
}
}
}
, complete: function (_file, _res) {
// var el = $(this.element);
// if (isStatus_FileUI3) {
// $("#lock").hide();
// $("#debug").html("");
// // for (var key in _res) {
// // $("#debug").append('' + "[" + key + "]" + _res[key] + '
');
// // }
// alert(_res);
// $("#debug").append(_res);
// $("#debug").show();
//
// if (callbackFileUI3) {
// callbackFileUI3 += "(el)";
// eval(callbackFileUI3);
// return;
// }
// }
}
, sending: function (_file) {
var el = $(this.element);
el.find(".dz-preview").remove();
isStatus_FileUI3 = true;
$("#lock").show();
}
, init: function () {
Dropzone.autoDiscover = false;
Dropzone.myAwesomeDropzone = false;
}
, error: function (_file, _error_msg) {
var el = $(this.element);
el.find(".dz-preview").remove();
alert(_error_msg);
isStatus_FileUI3 = false;
}
, dictDefaultMessage: ""
, dictInvalidFileType: "アップロードできるファイルを選択してください。[" + $(this).data("type") + "]"
});
$(this).find(".dz-message").remove();
$(this).css({ "min-height": "auto" });
});
}
function $CKEditorUI() {
$(".ckeditorUI").each(function () {
var msg = new Array;
if (!$(this).attr("id")) msg.push("CKEditor UI[id]を指定してください");
if (!$(this).data("extension")) msg.push("CKEditor UI[data-extension]を指定してください");
if (!$(this).data("name")) msg.push("CKEditor UI[data-name]を指定してください");
if (!$(this).data("col")) msg.push("CKEditor UI[data-col]を指定してください");
if (!$(this).data("index")) msg.push("CKEditor UI[data-index]を指定してください");
// if (!$(this).data("width")) msg.push("FILE UI[width]を指定してください");
// if (!$(this).data("height")) msg.push("FILE UI[height]を指定してください");
if (msg.length > 0) {
alert(msg.join("\n"));
$(this).css("border", "10px solid #f00");
return;
}
var uploadUrl = ajax_top + '/fileupload.ashx?idx=1&key1=' + $(this).data("index") + '&extension=' + $(this).data("extension") + '&datakey=DEPROG_CKE&width=' + (($(this).data("width")) ? $(this).data("width") : '') + '&height=' + (($(this).data("height")) ? $(this).data("height") : '');
CKEDITOR.replace($(this).attr("id"), {
extraPlugins: ['maximize', 'image2', 'uploadfile', 'uploadimage']
// 使用ボタン
, toolbarGroups: [
{ name: 'document', groups: ['mode', 'tools', 'document'] }
, { name: 'others', groups: ['others'] }
, { name: 'clipboard', groups: ['clipboard', 'undo'] }
, { name: 'paragraph', groups: ['list', 'indent', 'blocks', 'align', 'bidi', 'paragraph'] }
, { name: 'links', groups: ['links'] }
, { name: 'insert', groups: ['insert'] }
, '/'
, { name: 'styles', groups: ['styles'] }
, { name: 'basicstyles', groups: ['basicstyles', 'cleanup'] }
, { name: 'colors', groups: ['colors'] }
]
// 不要なボタンを削除
, removeButtons: 'Save,Flash,Iframe,PageBreak,NewPage,Language'
// ファイルアップロード
, filebrowserUploadUrl: uploadUrl
, uploadUrl: uploadUrl
, imageUploadUrl: uploadUrl
// オプション
, height: (($(this).data("height")) ? $(this).data("height") : "300")
, width: (($(this).data("width ")) ? $(this).data("width ") : "100%")
, allowedContent: true
, resizeEnabled: true
, enterMode: CKEDITOR.ENTER_BR
, shiftEnterMode: CKEDITOR.ENTER_P
});
setTimeout(function () {
$(".cke_chrome").css("border", "none");
$(".cke_top, .cke_contents, .cke_bottom").css("border", "1px solid #d1d1d1");
}, 500);
});
}
var oTableUI = new Object;
var oTableUIParms = new Object;
function $TableUI() {
$(".tableUI").each(function () {
var msg = new Array;
if (!$(this).attr("id")) msg.push("TABLE UI[id]を指定してください");
if (!$(this).data("extension")) msg.push("TABLE UI[data-extension]を指定してください");
if (!$(this).data("name")) msg.push("TABLE UI[data-name]を指定してください");
if (msg.length > 0) {
alert(msg.join("\n"));
$(this).css("border", "10px solid #f00");
return;
}
// table css setting
// "display","nowrap","table","table-hover","table-striped","table-bordered"
var lstCss = ["display", "nowrap", "table", "table-hover", "table-striped", "table-bordered"];
for (var idxCss = 0; idxCss < lstCss.length; idxCss++) {
if (!$(this).hasClass(lstCss[idxCss])) $(this).addClass(lstCss[idxCss]);
}
// column setting
var links = new Array;
var parms = new Array;
var parms2 = new Array;
var baseSort = new Array;
var parmsIdx = -1;
$(this).find("colgroup col").each(function () {
if ($(this).data("link")) {
// left join
var opt = new Object;
opt["name"] = $(this).data("name");
opt["col"] = $(this).data("col");
opt["from"] = $(this).data("from");
opt["from-col"] = $(this).data("from-col");
//create parm
links.push(opt);
} else {
parmsIdx += 1;
// sort (init drawing)
var opt = new Object;
opt["sCol"] = $(this).data("col"); //[COLUMN NAME], DUMMY
if ($(this).data("title")) opt["title"] = $(this).data("title");
if ($(this).data("width")) opt["width"] = $(this).data("width");
if ($(this).data("type")) opt["sType"] = $(this).data("type");
if ($(this).data("init") || $(this).data("init") == "0") opt["sInit"] = $(this).data("init");
if ($(this).data("sort")) opt["bSortable"] = true;
if ($(this).data("visible")) opt["bVisible"] = true;
if ($(this).data("search")) opt["search"] = true;
if ($(this).data("insert")) opt["add"] = true;
// JOIN 20210816
if ($(this).data("join")) opt["join"] = $(this).data("join");
// sort (init drawing)
if ($(this).data("sorted")) {
//opt["sSort"] = parmsIdx;
var oneSort = new Array;
oneSort.push(parmsIdx);
oneSort.push($(this).data("sorted"));
baseSort.push(oneSort);
}
//create parm
parms.push(opt);
parms2.push($(this).data());
}
});
// drawing
$(this).css({ "width": "100%", "tableLayout": "fixed" });
var psize = (($(this).data("psize")) ? $(this).data("psize") : "25");
oTableUIParms[$(this).attr("id")] = parms2;
if ($(this).data("auth") == "1") {
oTableUI[$(this).attr("id")] = ajax_select_cl($(this).attr("id"), $(this).data("extension"), $(this).data("name"), parms, links, $(this).data("dom"), psize, baseSort
, function (nRow, aData, iDataIndex, targetID) {
//base
$(nRow).attr("pid", aData["ID"]);
$('td', nRow).addClass("td2");
//custom function
var makeRow = $("#" + targetID).data("makerow");
if (makeRow) {
makeRow += "(nRow, aData, iDataIndex, targetID)";
eval(makeRow);
return;
}
//render
var idx = -1;
for (var parmsI = 0; parmsI < oTableUIParms[targetID].length; parmsI++) {
if (oTableUIParms[targetID][parmsI]["visible"]) {
idx++;
if (oTableUIParms[targetID][parmsI]["col"] == "ID") continue;
if (oTableUIParms[targetID][parmsI]["col"] == "DUMMY") continue;
var inputType = "text";
switch (oTableUIParms[targetID][parmsI]["type"]) {
case "int":
case "numeric":
inputType = "number";
break;
case "date":
inputType = "date";
break;
case "string":
default:
break;
}
if (oTableUIParms[targetID][parmsI]["modify"]) {
$('td:eq(' + String(idx) + ')', nRow).html('');
} else {
$('td:eq(' + String(idx) + ')', nRow).html('');
}
}
}
// command
if ($("#" + targetID).data("update")) {
$('td:eq(' + String(idx) + ')', nRow).append('');
}
if ($("#" + targetID).data("remove")) {
$('td:eq(' + String(idx) + ')', nRow).append('');
}
}
, function (oSettings, targetID) {
//completed
$(".otable-update.btn, .otable-remove.btn").css({ width: "auto", margin: "1px 3px" });
//custom function
var makeTable = $("#" + targetID).data("maketable");
if (makeTable) {
makeTable += "(oSettings, targetID)";
eval(makeTable);
return;
}
});
} else {
oTableUI[$(this).attr("id")] = ajax_select($(this).attr("id"), $(this).data("extension"), $(this).data("name"), parms, links, $(this).data("dom"), psize, baseSort
, function (nRow, aData, iDataIndex, targetID) {
//base
$(nRow).attr("pid", aData["ID"]);
$('td', nRow).addClass("td2");
//custom function
var makeRow = $("#" + targetID).data("makerow");
if (makeRow) {
makeRow += "(nRow, aData, iDataIndex, targetID)";
eval(makeRow);
return;
}
//render
var idx = -1;
for (var parmsI = 0; parmsI < oTableUIParms[targetID].length; parmsI++) {
if (oTableUIParms[targetID][parmsI]["visible"]) {
idx++;
if (oTableUIParms[targetID][parmsI]["col"] == "ID") continue;
if (oTableUIParms[targetID][parmsI]["col"] == "DUMMY") continue;
var inputType = "text";
switch (oTableUIParms[targetID][parmsI]["type"]) {
case "int":
case "numeric":
inputType = "number";
break;
case "date":
inputType = "date";
break;
case "string":
default:
break;
}
if (oTableUIParms[targetID][parmsI]["modify"]) {
$('td:eq(' + String(idx) + ')', nRow).html('');
} else {
$('td:eq(' + String(idx) + ')', nRow).html('');
}
}
}
// command
if ($("#" + targetID).data("update")) {
$('td:eq(' + String(idx) + ')', nRow).append('');
}
if ($("#" + targetID).data("remove")) {
$('td:eq(' + String(idx) + ')', nRow).append('');
}
}
, function (oSettings, targetID) {
//completed
$(".otable-update.btn, .otable-remove.btn").css({ width: "auto", margin: "1px 3px" });
//custom function
var makeTable = $("#" + targetID).data("maketable");
if (makeTable) {
makeTable += "(oSettings, targetID)";
eval(makeTable);
return;
}
});
}
// new data make button
if ($("#" + $(this).attr("id") + " tr.datatables-new").length > 0) {
var insertRow = $("#" + $(this).attr("id")).data("insertrow");
if (insertRow) {
insertRow += '(\'' + $(this).attr("id") + '\',\'0\')';
$("#" + $(this).attr("id") + " tr.datatables-new " + 'td').last().append('');
} else {
$("#" + $(this).attr("id") + " tr.datatables-new " + 'td').last().append('');
}
}
});
}
function oTable_Update(targetID, pid) {
var ht = new Object;
var msg = new Array;
for (var parmsI = 0; parmsI < oTableUIParms[targetID].length; parmsI++) {
if (oTableUIParms[targetID][parmsI]["visible"]) {
if (oTableUIParms[targetID][parmsI]["col"] == "ID") continue;
if (oTableUIParms[targetID][parmsI]["col"] == "DUMMY") continue;
if (!oTableUIParms[targetID][parmsI]["modify"]) continue;
ht[oTableUIParms[targetID][parmsI]["col"]] = GetValue($("#" + targetID + "_" + oTableUIParms[targetID][parmsI]["col"] + "_" + pid));
if (oTableUIParms[targetID][parmsI]["required"]) {
if (!ht[oTableUIParms[targetID][parmsI]["col"]]) {
msg.push("[" + oTableUIParms[targetID][parmsI]["title"] + "]を入力してください。");
$("#" + targetID + "_" + oTableUIParms[targetID][parmsI]["col"] + "_" + pid).css("backgroundColor", "#fcc");
$("#" + targetID + "_" + oTableUIParms[targetID][parmsI]["col"] + "_" + pid).focus(function () {
$(this).css("backgroundColor", "");
});
}
}
}
}
// var pp = new Array;
// for (var key in ht) {
// pp.push("[" + key + "]" + ht[key]);
// }
// alert("[pid]" + pid + "\n" + pp.join("\n"));
if (msg.length > 0) {
alert(msg.join("\n"));
return;
}
if ($("#" + targetID).data("auth") == "1") {
ajax_update_cl($("#" + targetID).data("extension"), $("#" + targetID).data("name"), ((pid == "0") ? "" : pid), GetValue($("#" + targetID + "_ACOUNT_" + pid)), GetValue($("#" + targetID + "_PASSWD_" + pid)), ht, false, function () {
//oTableUI[targetID].fnDraw(false);
oTableUI[targetID].draw(false);
Pop_Save();
if (pid == "0") oTable_ClearText(targetID, pid);
});
} else {
ajax_update($("#" + targetID).data("extension"), $("#" + targetID).data("name"), ((pid == "0") ? "" : pid), ht, false, function () {
//oTableUI[targetID].fnDraw(false);
oTableUI[targetID].draw(false);
Pop_Save();
if (pid == "0") oTable_ClearText(targetID, pid);
});
}
}
function oTable_ClearText(targetID, pid) {
for (var parmsI = 0; parmsI < oTableUIParms[targetID].length; parmsI++) {
if (oTableUIParms[targetID][parmsI]["visible"]) {
if (oTableUIParms[targetID][parmsI]["col"] == "ID") continue;
if (oTableUIParms[targetID][parmsI]["col"] == "DUMMY") continue;
$("#" + targetID + "_" + oTableUIParms[targetID][parmsI]["col"] + "_" + pid).val("").css("backgroundColor", "");
}
}
}
function oTable_Remove(targetID, pid) {
if (confirm("削除します")) {
if ($("#" + targetID).data("auth") == "1") {
ajax_remove_cl($("#" + targetID).data("extension"), $("#" + targetID).data("name"), pid, false, function () {
//oTableUI[targetID].fnDraw(false);
oTableUI[targetID].draw(false);
Pop_Remove();
});
} else {
ajax_remove($("#" + targetID).data("extension"), $("#" + targetID).data("name"), pid, false, function () {
//oTableUI[targetID].fnDraw(false);
oTableUI[targetID].draw(false);
Pop_Remove();
});
}
}
}
function $FormUI() {
$Form_Init();
$CKEditorUI();
$FileUI();
$FileUI2();
$FileUI3();
$(".formUI").each(function () {
// validate
var msg = new Array;
if (!$(this).data("uid")) msg.push("Form UI[data-uid]を指定してください");
if (!$(this).data("extension")) msg.push("Form UI[data-extension]を指定してください");
if (!$(this).data("name")) msg.push("Form UI[data-name]を指定してください");
if (msg.length > 0) {
alert(msg.join("\n"));
$(this).css("border", "10px solid #f00");
return;
}
// column list
var arrCols = new Array;
$(this).find("*").each(function () {
if ($(this).data("col")) arrCols.push($(this).data("col"));
});
// get value
var rowItem;
if ($(this).data("auth") == "1") {
rowItem = ajax_get_cl($(this).data("extension"), $(this).data("name"), $(this).data("uid"), arrCols);
} else {
rowItem = ajax_get($(this).data("extension"), $(this).data("name"), $(this).data("uid"), arrCols);
}
// make element
if (rowItem) {
for (var rowI = 0; rowI < arrCols.length; rowI++) {
var el = $(this).find('[data-col="' + arrCols[rowI] + '"]');
if (el) {
switch (el.data("mode")) {
case "cke":
CKEDITOR.instances[el.data("name") + "_" + el.data("col") + "_" + el.data("index")].setData(rowItem[arrCols[rowI]]);
break;
case "file":
// NA
break;
default:
el.val(rowItem[arrCols[rowI]]);
el.data("base", rowItem[arrCols[rowI]]);
break;
}
}
}
if ($(this).data("autosave") == "1") {
setInterval(Form_Save, 60000);
}
} else {
for (var rowI = 0; rowI < arrCols.length; rowI++) {
var el = $(this).find('[data-col="' + arrCols[rowI] + '"]');
if (el) {
switch (el.data("mode")) {
case "cke":
CKEDITOR.instances[el.data("name") + "_" + el.data("col") + "_" + el.data("index")].setData("");
CKEDITOR.instances[el.data("name") + "_" + el.data("col") + "_" + el.data("index")].config.readOnly = true;
break;
case "file":
// NA
break;
default:
el.val("");
el.prop('disabled', true);
break;
}
}
}
Pop_Message("データが存在しません", true);
}
});
}
function $Form_Init() {
$(".formUI").each(function () {
if ($(this).data("name")) {
$(this).find(".ckeditorUI").data({
"extension": $(this).data("extension")
, "name": $(this).data("name")
, "mode": "cke"
});
$(this).find(".ckeditorUI").each(function () {
$(this).attr("id", $(this).data("name") + "_" + $(this).data("col") + "_" + $(this).data("index"));
});
$(this).find(".fileUI").data({
"extension": $(this).data("extension")
, "name": $(this).data("name")
, "uid": $(this).data("uid")
, "mode": "file"
});
$(this).find(".fileUI2").data({
"uid": $(this).data("uid")
, "mode": "file"
});
}
});
}
function Form_Save(isManual) {
$(".formUI").each(function () {
// column list
var arrCols = new Array;
$(this).find("*").each(function () {
if ($(this).data("col")) arrCols.push($(this).data("col"));
});
// change check
var isUpdate = false;
for (var rowI = 0; rowI < arrCols.length; rowI++) {
if ($(this).find('[data-col="' + arrCols[rowI] + '"]').val() != $(this).find('[data-col="' + arrCols[rowI] + '"]').data("base")) {
isUpdate = true;
break;
}
}
if (isUpdate) {
for (var rowI = 0; rowI < arrCols.length; rowI++) {
var el = $(this).find('[data-col="' + arrCols[rowI] + '"]');
if (el) {
switch (el.data("mode")) {
case "cke":
var ckeVal = CKEDITOR.instances[el.data("name") + "_" + el.data("col") + "_" + el.data("index")].getData();
htUpdate[arrCols[rowI]] = ckeVal;
el.data("base", ckeVal);
break;
case "file":
// NA
break;
default:
htUpdate[arrCols[rowI]] = el.val();
el.data("base", el.val());
break;
}
}
}
ajax_update($(this).data("extension"), $(this).data("name"), (($(this).data("uid")) ? $(this).data("uid") : ""), htUpdate, false, function (res) {
if (isManual) {
Pop_Save();
} else {
Pop_AutoSave();
}
});
}
});
}
/************************
** common.db.cl.js [Write file text]
************************/
/**
Deprog Tool Kit 2015/12/01
CustomLogin
**/
function ajax_get_cl(mkey, loginkey, uid, arrCols) {
// require parms
var parms = new Object;
parms["mkey"] = mkey;
parms["loginkey"] = loginkey;
//use columns
if (arrCols) {
for (i = 0; i < arrCols.length; i++) {
parms["Col_" + String(i)] = arrCols[i];
}
}
//one data
parms["sCol_0"] = "ID";
parms["sVal_0"] = uid;
var ret = new Object;
$.ajax({
url: ajax_top + '/list_cl.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: false
, data: parms
, success: function (res) {
if (res["result"] === "success") {
if (res["data"].length > 0) {
ret = res["data"][0];
} else {
ret = null;
}
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
return ret;
}
function ajax_list_cl(mkey, loginkey, arrCols, htCols, sort, async, success, error, isOne) {
if (async) async = true;
else async = false;
if (arrCols) {
// require parms
var parms = new Object;
parms["mkey"] = mkey;
parms["loginkey"] = loginkey;
//check parms
for (i = 0; i < arrCols.length; i++) {
parms["Col_" + String(i)] = arrCols[i];
}
//search column
if (htCols) {
var idx = 0;
for (var key in htCols) {
parms["sCol_" + String(idx)] = key;
parms["sVal_" + String(idx)] = htCols[key];
idx++;
}
}
//sort
if (sort) parms["sort"] = sort;
//isOne
if (isOne) parms["isOne"] = "1";
if (success) {
$.ajax({
url: ajax_top + '/list_cl.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] === "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
} else {
alert("引数[arrCols]を指定してください。");
}
}
function ajax_select_cl(targetID, mkey, loginkey, parms, links, sDom, iDisplayLength, aaSorting, fnCreatedRow, fnDrawCallback, scrollY, scrollHeight) {
//validation
var msg = new Array;
if (!targetID) msg.push("引数[targetID]を指定してください。");
if ($('#' + targetID).length === 0) msg.push("指定したテーブルが存在しません。");
if (!loginkey) msg.push("引数[loginkey]を指定してください。");
if (!parms) msg.push("引数[parms]を指定してください。");
if (!sDom) sDom = '<"H"lfip>t<"F"ip>';
if (!iDisplayLength) iDisplayLength = 25;
if (msg.length > 0) {
alert(msg.join("\n"));
return false;
}
//default
for (i = 0; i < parms.length; i++) {
if (!parms[i]["title"]) parms[i]["title"] = "COL_" + String(i);
if (!parms[i]["width"]) parms[i]["width"] = "";
if (!parms[i]["sCol"]) parms[i]["sCol"] = "";
if (!parms[i]["sType"]) parms[i]["sType"] = "";
if (!parms[i]["sInit"]) {
if (parms[i]["sInit"] == "0") {
//ZERO
} else {
parms[i]["sInit"] = "";
}
}
if (!parms[i]["sWhere"]) parms[i]["sWhere"] = "";
if (!parms[i]["sBeetween"]) parms[i]["sBeetween"] = "";
if (!parms[i]["sOr"]) parms[i]["sOr"] = "";
else parms[i]["sOr"] = "1";
if (!parms[i]["sSort"]) parms[i]["sSort"] = "";
if (!parms[i]["bSortable"]) parms[i]["bSortable"] = false;
if (!parms[i]["bVisible"]) parms[i]["bVisible"] = false;
if (!parms[i]["search"]) parms[i]["search"] = false;
if (!parms[i]["add"]) parms[i]["add"] = false;
// JOIN 20210816
if (!parms[i]["join"]) parms[i]["join"] = "";
// JOIN 20210816
}
//table clear
if (targetID) $("#" + targetID).html("");
//table options
var isSearch = false;
var isAdd = false;
for (i = 0; i < parms.length; i++) {
if (parms[i]["search"]) isSearch = true;
if (parms[i]["add"]) isAdd = true;
}
//make title
var thead_title = $("
");
var tfoot_title = $("
");
var thead_2 = $("
");
var thead_3 = $("
");
for (i = 0; i < parms.length; i++) {
//input type
var inputType = "text";
switch (parms[i]["sType"]) {
case "int":
case "numeric":
parms[i]["sType"] = "numeric";
inputType = "number";
break;
case "date":
parms[i]["sType"] = "";
inputType = "date";
break;
case "string":
default:
parms[i]["sType"] = "";
break;
}
//title
if ((!parms[i]["width"]) || (parms[i]["width"] === "auto") || (!parms[i]["bVisible"])) {
thead_title.append('' + parms[i]["title"] + ' | ');
tfoot_title.append('' + parms[i]["title"] + ' | ');
} else {
thead_title.append('' + parms[i]["title"] + ' | ');
tfoot_title.append('' + parms[i]["title"] + ' | ');
}
//search
if (isSearch) {
if (parms[i]["sCol"]) {
switch (parms[i]["search"]) {
case "auto":
case true:
thead_2.append(' | ');
break;
default:
thead_2.append(' | ');
break;
}
} else {
thead_2.append(' | ');
}
}
//add
if (isAdd) {
if (parms[i]["sCol"]) {
switch (parms[i]["add"]) {
case "auto":
case true:
thead_3.append(' | ');
break;
default:
thead_3.append(' | ');
break;
}
} else {
thead_3.append(' | ');
}
}
}
//bulid table layout
var thead = $("").append(thead_title);
var tbody = $("").append('データ受信中... |
');
var tfoot = $("").append(tfoot_title);
if (isSearch) tfoot.append(thead_2);
if (isAdd) tfoot.append(thead_3);
//paging off 20220524
if ($('#' + targetID).data("pagingoffH") != "1") $('#' + targetID).append(thead);
$('#' + targetID).append(tbody);
if ($('#' + targetID).data("pagingoffF") != "1") $('#' + targetID).append(tfoot);
// $('#' + targetID).append(thead).append(tbody).append(tfoot);
//ajax path and post data
var ajax_data = new Object;
var ajax_path = ajax_top + '/select_cl.ashx';
ajax_data['mkey'] = mkey;
ajax_data['loginkey'] = loginkey;
for (i = 0; i < parms.length; i++) {
ajax_data["sCol_" + String(i)] = parms[i]["sCol"];
ajax_data["sType_" + String(i)] = parms[i]["sType"];
ajax_data["sInit_" + String(i)] = parms[i]["sInit"];
ajax_data["sWhere_" + String(i)] = parms[i]["sWhere"];
ajax_data["sBeetween_" + String(i)] = parms[i]["sBeetween"];
ajax_data["sOr_" + String(i)] = parms[i]["sOr"];
ajax_data["sSort_" + String(i)] = parms[i]["sSort"];
// JOIN 20210816
ajax_data["join_" + String(i)] = parms[i]["join"];
// JOIN 20210816
}
if (links) {
for (i = 0; i < links.length; i++) {
ajax_data["lnkName_" + String(i)] = links[i]["name"];
ajax_data["lnkCol_" + String(i)] = links[i]["col"];
ajax_data["lnkFrom_" + String(i)] = links[i]["from"];
ajax_data["lnkFromCol_" + String(i)] = links[i]["from-col"];
}
}
//aoColumns
var aoColumns = new Array;
for (i = 0; i < parms.length; i++) {
aoColumns.push({ sType: parms[i]["sType"], bSortable: parms[i]["bSortable"], bVisible: parms[i]["bVisible"] });
}
//bulid datatables
var oTable;
$('#' + targetID).removeClass("tableUI");
oTable = $('#' + targetID).DataTable({
"sDom": sDom
, "buttons": ['excel', 'pdf', 'print']
, "bAutoWidth": false
, "iDisplayLength": iDisplayLength
, "aaSorting": aaSorting
, "aoColumns": aoColumns
// use ajax
, "bProcessing": true
, "bDeferRender": true
, "bServerSide": true
, "ajax": {
url: ajax_path
, type: 'POST'
, data: ajax_data
, dataSrc: function (json) {
if (json["custom-error"] == "1") {
alert("[DataTable]\n" + targetID + "\n[システム障害]\n" + json["custom-error-message"] + "\n[指定した列情報]\n" + json["custom-error-columns"]);
return true;
} else {
// var val = new Array;
// for (var key in json) {
// val.push("["+key+"]"+json[key]);
// }
return json["aaData"];
}
}
}
// make row
, "fnCreatedRow": function (nRow, aData, iDataIndex) {
var ret = new Object;
for (i = 0; i < parms.length; i++) {
ret[parms[i]["sCol"].replace(".", "_")] = aData[i];
ret[i] = aData[i];
}
aData = ret;
fnCreatedRow(nRow, aData, iDataIndex, targetID);
}
// complete
, "fnDrawCallback": function (oSettings) {
$(".datatables-top td, .datatables-new td").removeClass("ui-state-default");
if (scrollY) $(window).trigger("resize");
$(".dataTables_filter").hide();
$(".dataTables_empty").width($('#' + targetID).width());
fnDrawCallback(oSettings, targetID);
}
});
$('#' + targetID + " .datatables-top").appendTo($('#' + targetID + " thead"));
$('#' + targetID + " .datatables-new").appendTo($('#' + targetID + " thead"));
// if (!scrollY) {
// $('#' + targetID + " tfoot").remove();
// }
//event
if (scrollY) {
$(window).resize(function () {
windows_table_fix(targetID, scrollHeight);
});
$("#" + targetID + " tbody").scroll(table_height);
}
//base search
for (i = 0; i < parms.length; i++) {
if (parms[i]["sInit"]) {
SearchColSetting(oTable, i, parms[i]["sInit"]);
} else if (parms[i]["sInit"] == "0") {
SearchColSetting(oTable, i, parms[i]["sInit"]);
}
}
//search columns
for (i = 0; i < parms.length; i++) {
//search
if (isSearch) {
if (parms[i]["sCol"]) {
switch (parms[i]["search"]) {
case "auto":
case true:
$('#' + targetID + '_' + parms[i]["sCol"].replace(".", "_") + '_search input').keyup(function (e) {
if (e.keyCode === 13) {
$('#' + targetID + " .datatables-top input").each(function () {
if ($(this).attr("column_index")) {
SearchColSetting(oTable, $(this).attr("column_index"), $(this).val());
}
});
oTable.draw();
}
});
break;
default:
break;
}
}
}
}
//complete
return oTable;
}
function ajax_update_cl(mkey, loginkey, uid, acount, passwd, htCols, async, success, error) {
if (async) async = true;
else async = false;
if (htCols) {
// require parms
var parms = new Object;
parms["uid"] = uid;
parms["mkey"] = mkey;
parms["loginkey"] = loginkey;
parms["acount"] = acount;
parms["passwd"] = passwd;
//check column
if (htCols) {
var idx = 0;
for (var key in htCols) {
parms["Col_" + String(idx)] = key;
parms["Val_" + String(idx)] = htCols[key];
idx++;
}
}
if (success) {
$.ajax({
url: ajax_top + '/update_cl.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] === "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
} else {
alert("引数[htCols]を指定してください。");
}
}
function ajax_remove_cl(mkey, loginkey, uid, async, success, error) {
if (async) async = true;
else async = false;
var parms = new Object;
parms["uid"] = uid;
parms["mkey"] = mkey;
parms["loginkey"] = loginkey;
if (success) {
$.ajax({
url: ajax_top + '/remove_cl.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: async
, data: parms
, success: function (res) {
if (res["result"] === "success") {
success(res);
} else alert(res["msg"]);
}
, error: function (XMLHttpRequest, textStatus, errorThrown) {
if (error) {
error(XMLHttpRequest, textStatus, errorThrown);
} else {
ajax_error(XMLHttpRequest, textStatus, errorThrown);
}
}
});
} else {
alert("引数[success(Callback関数)]を指定してください。");
}
}
function ajax_islogin(key, mkey) {
// require parms
var parms = new Object;
parms["key"] = key;
parms["mkey"] = mkey;
parms["action"] = "islogin";
var islogin = false;
$.ajax({
url: ajax_top + '/acount.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: false
, data: parms
, success: function (res) {
if (res["result"] === "success") {
if (res["data"]) {
islogin = true;
} else {
islogin = false;
}
} else alert(res["msg"]);
}
, error: ajax_error
});
// login check
return islogin;
}
function ajax_loginuser(key, mkey) {
// require parms
var parms = new Object;
parms["key"] = key;
parms["mkey"] = mkey;
parms["action"] = "get";
var ret = new Object;
$.ajax({
url: ajax_top + '/acount.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: false
, data: parms
, success: function (res) {
if (res["result"] === "success") {
if (res["data"]) {
ret = res["data"];
}
} else alert(res["msg"]);
}
, error: ajax_error
});
// data
return ret;
}
function ajax_login(uid, pass, key, mkey, url, sucess, fail) {
// require parms
var parms = new Object;
parms["uid"] = uid;
parms["pass"] = pass;
parms["key"] = key;
parms["mkey"] = mkey;
parms["action"] = "login";
Loading_Start();
$.ajax({
url: ajax_top + '/acount.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: false
, data: parms
, success: function (res) {
if (res["result"] === "success") {
if (res["data"]) {
if ($("#login_iframe").length === 0) {
$('', {
id: "login_iframe"
, style: "display:none;"
, src: ajax_top + "/acount.aspx?uid=" + uid + "&pass=" + pass + "&key=" + key + "&mkey=" + mkey + "&action=" + parms["action"]
}).appendTo('body');
} else {
$("#login_iframe").attr("src", ajax_top + "/acount.aspx?uid=" + uid + "&pass=" + pass + "&key=" + key + "&mkey=" + mkey + "&action=" + parms["action"]);
}
$("#login_iframe").on("load", function () {
if (sucess) {
sucess(res);
} else if (url) {
location.href = url;
} else {
//NA
}
});
} else {
Loading_Exit();
if (fail) fail(res);
}
} else {
Loading_Exit();
alert(res["msg"]);
}
}
, error: ajax_error
});
}
function ajax_logout(key, mkey, url, sucess) {
// require parms
var parms = new Object;
parms["key"] = key;
parms["mkey"] = mkey;
parms["action"] = "logout";
$.ajax({
url: ajax_top + '/acount.ashx'
, type: 'POST'
, cache: false
, dataType: 'json'
, async: false
, data: parms
, success: function (res) {
if (res["result"] === "success") {
if ($("#login_iframe").length === 0) {
$('', {
id: "login_iframe"
, style: "display:none;"
, src: ajax_top + "/acount.aspx?key=" + key + "&mkey=" + mkey + "&action=" + parms["action"]
}).appendTo('body');
} else {
$("#login_iframe").attr("src", ajax_top + "/acount.aspx?key=" + key + "&mkey=" + mkey + "&action=" + parms["action"]);
}
$("#login_iframe").on("load", function () {
if (sucess) {
sucess(res);
} else if (url) {
location.href = url;
} else {
//NA
}
});
} else alert(res["msg"]);
}
, error: ajax_error
});
}